As of Python version 3.7, dictionaries are ordered. The important thing is that its fast across a wide range of circumstances: it doesnt get significantly slower when the dictionary has a lot of stuff in it, or when the keys or values are big values. If
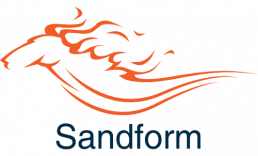